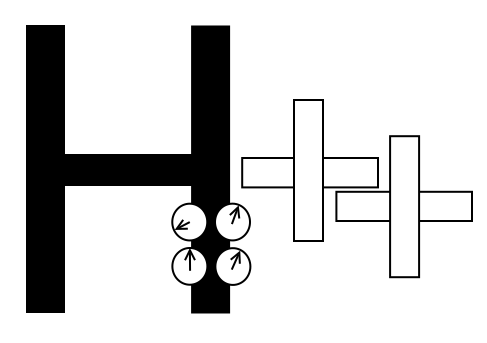 |
A parallel C++ Library for Simulations in the Heisenberg Model
|
Go to the documentation of this file.
11 #include <cuda_runtime.h>
12 #include <thrust/host_vector.h>
13 #include <thrust/device_vector.h>
43 Grid(
const short int spatialDimensions,
const dim3 gridSize) :
mDim(dim),
mGridSize(gridSize)
45 mGridData.resize(gridSize.x*gridSize.y*gridSize.z);
61 mGridData.resize(gridSize.x*gridSize.y*gridSize.z);
97 void setSpin(
const dim3 index,
const T spin)
void calcNeighbourTable()
generates a table of the neighbouring points of each spin and stores it
A struct containing six neighbour inidices of a spin in three dimensions.
void setSpin(const dim3 index, const T spin)
T getSpin(const dim3 index) const
void setGridSize(const dim3 gridSize)
sets grid size nad resizes grid
thrust::host_vector< T > mGridData
void setNeighbourTable(const thrust::host_vector< NB > &neighbourTable)
void setDim(const short int dim)
thrust::host_vector< T > getGridData() const
Grid(const short int spatialDimensions, const dim3 gridSize)
constructs a grid and allocates memory
thrust::host_vector< NB > getNeighbourTable() const
void hotStart()
initializes all spins randomly between 0 and 2*pi
void coldStart()
initializes all spins as up, i.e. mGrid.x[...] = 0.5*pi and mGrid.y[...] = 0
A class storing spin values represented by two angles.
void setGridData(const thrust::host_vector< T > &gridData)
A class storing spin values as a grid and certain meta information.
herr_t saveGrid(const std::string path)
saves grid as hdf5 file
dim3 mGridSize
copies grid and table to device
NB getNeighbours(const dim3 index) const
thrust::host_vector< NB > mNeighbourTable