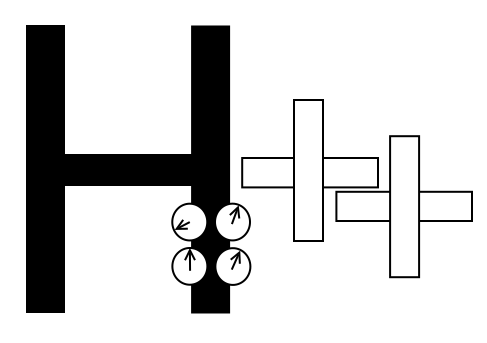 |
A parallel C++ Library for Simulations in the Heisenberg Model
|
Go to the documentation of this file.
4 #include <thrust/reduce.h>
5 #include <thrust/execution_policy.h>
18 mGrid = std::make_shared<Grid<short int>>(grid);
29 std::shared_ptr<Grid<short int>>
mGrid{};
65 mGrid = std::make_shared<Grid<short int>>(grid);
75 std::shared_ptr<Grid<short int>>
mGrid{};
107 mGrid = std::make_shared<Grid<short int>>(grid);
119 std::shared_ptr<Grid<short int>>
mGrid{};
154 template <
typename T> T
mean(
const std::vector<T> &o);
163 template <
typename T> T
covFunc(
const size_t t,
const std::vector<T> &o);
171 template <
typename T> T
intAuto(
const std::vector<T> &o);
179 template <
typename T> T
error(
const std::vector<T> &o);
188 template <
typename T> T
blockingError(
const std::vector<T> &o,
const size_t block_number);
199 template <
typename T> T
bootstrapError(
const std::vector<T> &o,
const size_t sample_size,
const size_t sample_number,
const T tau);
208 template <
typename T> T
errorProp(
const std::vector<T> &o,
const T tau);
217 template <
typename T> T
errorPropEnergy(
const std::vector<T> &energies,
const T beta,
const T tau,
const size_t V);
234 template <
typename T>
size_t d2i(
const T d);
246 template <
typename T>
void removeCorr(
const std::vector<T> &o);
257 template <
typename T> T
specificHeat(
const std::vector<T> &energies,
const T beta,
const size_t V);
268 template <
typename T> T
magnSusz(
const std::vector<T> &magn,
const T beta,
const size_t V);
270 using Models = std::vector<std::unique_ptr<HB::Model>>;
std::shared_ptr< Grid< short int > > mGrid
void simulate(const Ising &model)
performs a production-run.
float metropolisSweep(const M &model)
performs one Monte-Carlo step, updating the entire grid associated with a certain model and calculate...
void operator()(const M &model) const
T specificHeat(const std::vector< T > &energies, const T beta, const size_t V)
computes the specific heat per Volume as secondary quantity for a given vector of energies
T errorProp(const std::vector< T > &o, const T tau)
calculates the error based on error propagation of a standard vector
T mean(const std::vector< T > &o)
calculates the mean value of a standard vector
MetropolisSimulationQt(const Grid< short int > &grid, const float beta)
T intAuto(const std::vector< T > &o)
calculates the integrated autocorrelation time of a standard vector
float metropolisSweep(const Ising &model)
performs one Monte-Carlo step, updating the entire grid associated with a certain model and calculate...
void removeCorr(const std::vector< T > &o)
removes correlation from a standard vector
std::vector< float > mEnergies
std::vector< float > mMagnetization
T errorPropEnergy(const std::vector< T > &energies, const T beta, const T tau, const size_t V)
calculates the statistical error on energies using error propagation
T errorPropMagnetization(const std::vector< T > &magn, const T beta, const T tau, const size_t V)
calculates the statistical error on magnetizations (magn) using error propagation
std::shared_ptr< Grid< short int > > mGrid
void operator()(const Ising &model) const
void simulate(const M &model)
performs a production-run.
void operator()(const M &model) const
T blockingError(const std::vector< T > &o, const size_t block_number)
calculates the blocking error of a standard vector
std::shared_ptr< Grid< short int > > mGrid
std::vector< float > mEnergies
void simulate(const Models &models)
MetropolisSimulation(const Grid< short int > &grid, const float beta, const size_t steps)
void simulate(const M &model)
Performs a production-run via simulated annealing.
T bootstrapError(const std::vector< T > &o, const size_t sample_size, const size_t sample_number, const T tau)
calculates the bootstrap error of a standard vector
A class storing spin values as a grid and certain meta information.
std::vector< std::unique_ptr< HB::Model > > Models
T covFunc(const size_t t, const std::vector< T > &o)
calculates the covariance function up to a certain index of a standard vector
std::vector< float > mMagnetization
T magnSusz(const std::vector< T > &magn, const T beta, const size_t V)
computes the magnetic suszeptibility per Volume as secondary quantity for a given vector of magnetiza...
size_t d2i(const T d)
rounds a floating point value to an integer
SimulatedAnnealing(const Grid< short int > &grid, const float beta, const size_t steps, const float3 temperatureSteps)
T error(const std::vector< T > &o)
calculates the standard error (standard deviation) of a standard vector